앞에서 MongoDB를 Cloud 환경에서 활용하기 위한 준비를 해보았습니다. 여차하면 로컬 것을 쓰는걸로~
https://learn-and-give.tistory.com/116
[MDN/Express] 04.Express Tutorial Part 3: Using a Database (with Mongoose) (1)
앞에서 자동 생성 된 프로젝트의 구조를 살펴보았습니다. https://learn-and-give.tistory.com/115 [MDN/Express] 03.Express Tutorial: The Local Library website(2) 앞에서 동네 도서관 프로젝트를 위한 기본 코드 생성까지
learn-and-give.tistory.com
Defining and creating models
모델은 Schema 인터페이스를 사용하여 정의합니다. 모델 정의 외에도 여러가지 있는데 차차 알아보기로 하고.
스키마는 mongoose.model()이라는 메쏘드로 컴파일이 되고, 모델이 생성되면 이것으로 검색/생성/업데이트/삭제 등을 할 수 있습니다.
Defining schemas
스키마 정의는 아래의 아주 간단한 코드로 기본적인 내용은 파악 할 수 있을 것 같습니다.
//Require Mongoose
var mongoose = require('mongoose');
//Define a schema
var Schema = mongoose.Schema;
var SomeModelSchema = new Schema({
a_string: String,
a_date: Date
});
위의 모델은 속성이 두개 뿐이지만 도서관 데이터에는 좀 더 많은 속성들이 사용될 것 입니다.
Creating a model
모델의 생성도 간단한 코드로 한번 살펴 보겠습니다. 첫번째 인자가 모델의 이름입니다.
// Define schema
var Schema = mongoose.Schema;
var SomeModelSchema = new Schema({
a_string: String,
a_date: Date
});
// Compile model from schema
var SomeModel = mongoose.model('SomeModel', SomeModelSchema );
스키마 타입 (필드)
모델의 스키마는 여러가지 타입의 여러 개의 속성을 가질 수 있습니다. 아래와 같이 다양한 속성을 가질 수 있어요.
var schema = new Schema(
{
name: String,
binary: Buffer,
living: Boolean,
updated: { type: Date, default: Date.now },
age: { type: Number, min: 18, max: 65, required: true },
mixed: Schema.Types.Mixed,
_someId: Schema.Types.ObjectId,
array: [],
ofString: [String], // You can also have an array of each of the other types too.
nested: { stuff: { type: String, lowercase: true, trim: true } }
})
Mongoose에서 사용 가능한 스키마 타입은 별도 자료를 참고 합니다.
https://mongoosejs.com/docs/schematypes.html
Mongoose v7.2.2: SchemaTypes
SchemaTypes handle definition of path defaults, validation, getters, setters, field selection defaults for queries, and other general characteristics for Mongoose document properties. You can think of a Mongoose schema as the configuration object for a Mon
mongoosejs.com
몇 가지 특별한 사항을 기억 해 둘 필요가 있습니다.
- ObjectId : 이 ID는 DB 내에서 부여되는 식별 정보입니다. 그래서, 다른 데이터와 구별되는 식별 정보로 활용 될 수 있습니다.
- Mixed : 임의의 스키마 타입이며 어떠한 타입도 될 수 있습니다. 구조체 같은 것일까요??
- [] : 자바스크립트의 배열처럼 사용 할 수 있습니다.
위의 샘플을 보면, min/max, lowercase 뭐 이런 여러가지 특별한 옵션도 활용 가능합니다.
...
...
서비스를 만들려면 DB를 잘 사용해야 하기합니다만, 이거 너무 DB에 대한 내용이 많네요.
아래 토픽들은 요런게 있나보다 정도 생각 해 두고, 필요할 때 찾아보는걸로~
...
Validation
Virtual properties
Methods and query helpers
Using models
Creating and modifying documents
Searching for records
Working with related documents — population
코드를 보고 파악 할 수 있습니다.
One schema/model per file
요건 좀 중요한 내용 같은데, 권장 사항이지 필수는 아닌 것 같네요.
Setting up the MongoDB database
Mongoose를 사용하는 방법은 대충 알아봤다 치고~, cloud 기반으로 무료로 DB를 사용 할 수 있는 mLab을 기반으로 이후 내용이 진행 됩니다.(뭘 또 이렇게 새로운 걸 막...)
그런데, 접속을 해 보니 사용 할 수 없네요. @_@a...
음... 몇일전에는 MongoDB Atlas 기반의 내용이었던 것 같은데.. MongoDB Atlas의 사용법이 뒤에 나오니 나중에 봐야지 하고 생각 했었던 기억이 있는데... 다른 곳에서 본 것인지 긴가민가 하네요.
일단 mLab은 Skip하고 고고씽~
Install Mongoose
이제 다시 시작 해 보겠습니다. 먼저 몽구스 설치~ (앞에서 하지 않았었나??)
npm install mongoose --save
C:\Study\mdn_express_node\express-locallibrary-tutorial>npm install mongoose --save
added 8 packages, and audited 182 packages in 3s
13 packages are looking for funding
run `npm fund` for details
7 vulnerabilities (2 low, 5 high)
To address issues that do not require attention, run:
npm audit fix
To address all issues, run:
npm audit fix --force
Run `npm audit` for details.
C:\Study\mdn_express_node\express-locallibrary-tutorial>
앞에서 프로젝트 생성기로 만든 프로젝트로 돌아가서 mongoose를 사용하는 코드를 넣겠습니다. app 생성 코드 다음에 추가를 하라고 하네요.
...
var indexRouter = require('./routes/index');
var usersRouter = require('./routes/users');
var app = express();
// 여기부터
//Set up mongoose connection
var mongoose = require('mongoose');
var mongoDB = 'insert_your_database_url_here';
mongoose.connect(mongoDB);
mongoose.Promise = global.Promise;
var db = mongoose.connection;
db.on('error', console.error.bind(console, 'MongoDB connection error:'));
//여기까지
// view engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'pug');
...
URL을 넣는 부분을 mLab에서 확인한 것을 넣으라고 하는데, MongDB Atlas의 Connect를 눌러서 확인한 창에 있던 코드 내용 중에 URL이 있었습니다.
그 내용을 넣어 보겠습니다. 계정/암호/DB이름 등등은 자기 것을 적절히 써야겠죠. URL은 다르겠지만 요런 형식~
//Set up mongoose connection
var mongoose = require('mongoose');
var mongoDB = "mongodb+srv://<계정>:<password>@<DB>.q63vkgw.mongodb.net/?retryWrites=true&w=majority";
mongoose.connect(mongoDB);
이제 모델을 만들어야 하는데....
작성한 글은 길지 않지만 살펴본 글은 꽤 길어서 일단 여기까지...
피곤...
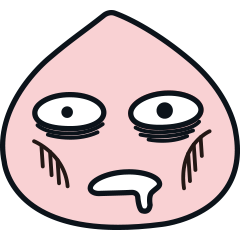
'공허의 유산 > 사상의 도구' 카테고리의 다른 글
Python 개발 환경 구축 (0) | 2023.08.27 |
---|---|
MariaDB와 HeidiSQL (0) | 2023.07.23 |
[MDN/Express] 04.Express Tutorial Part 3: Using a Database (with Mongoose) (1) (0) | 2023.06.01 |
[MDN/Express] 03.Express Tutorial: The Local Library website(2) (0) | 2023.05.28 |
[MDN/Express] 02.Express Tutorial: The Local Library website(1) (2) | 2023.05.27 |
댓글